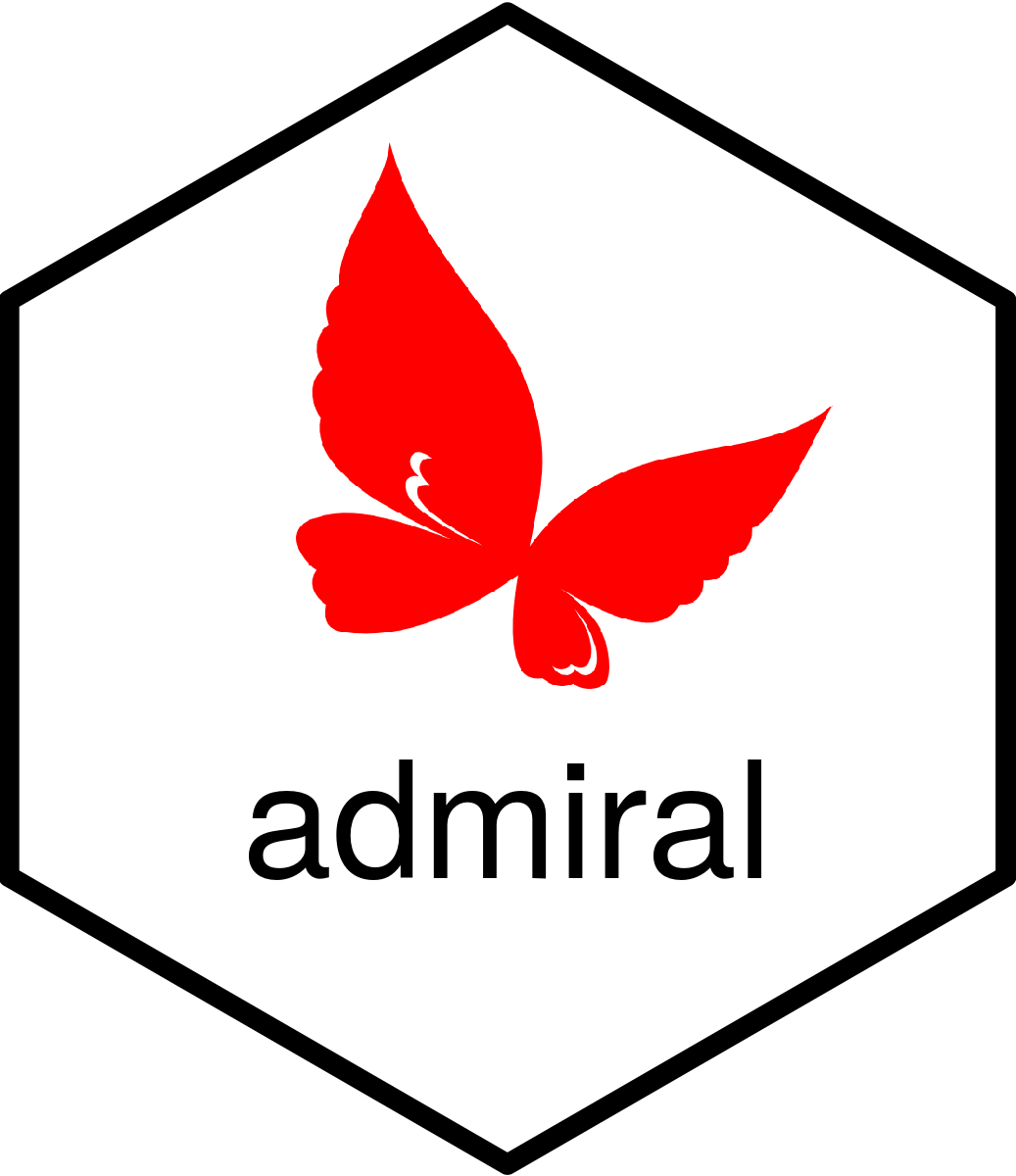
Derive First or Last Datetime from Multiple Sources
Source:R/derive_var_extreme_date.R
derive_var_extreme_dtm.Rd
Add the first or last datetime from multiple sources to the dataset, e.g.,
the last known alive datetime (LSTALVDTM
).
Usage
derive_var_extreme_dtm(
dataset,
new_var,
...,
source_datasets,
mode,
subject_keys = get_admiral_option("subject_keys")
)
Arguments
- dataset
Input dataset
The variables specified by
subject_keys
are required.- new_var
Name of variable to create
- ...
Source(s) of dates. One or more
date_source()
objects are expected.- source_datasets
A named
list
containing datasets in which to search for the first or last date- mode
Selection mode (first or last)
If
"first"
is specified, the first date for each subject is selected. If"last"
is specified, the last date for each subject is selected.Permitted Values:
"first"
,"last"
- subject_keys
Variables to uniquely identify a subject
A list of expressions where the expressions are symbols as returned by
exprs()
is expected.
Details
The following steps are performed to create the output dataset:
For each source dataset the observations as specified by the
filter
element are selected and observations wheredate
isNA
are removed. Then for each patient the first or last observation (with respect todate
andmode
) is selected.The new variable is set to the variable specified by the
date
element. If this is a date variable (rather than datetime), then the time is imputed as"00:00:00"
.The variables specified by the
traceability_vars
element are added.The selected observations of all source datasets are combined into a single dataset.
For each patient the first or last observation (with respect to the new variable and
mode
) from the single dataset is selected and the new variable is merged to the input dataset.
See also
date_source()
, derive_var_extreme_dt()
,
derive_vars_merged()
ADSL Functions that returns variable appended to dataset:
derive_var_age_years()
,
derive_var_dthcaus()
,
derive_var_extreme_dt()
,
derive_vars_aage()
,
derive_vars_period()
Examples
library(dplyr, warn.conflicts = FALSE)
library(admiral.test)
data("admiral_dm")
data("admiral_ae")
data("admiral_lb")
data("admiral_adsl")
# derive last known alive datetime (LSTALVDTM)
ae_start <- date_source(
dataset_name = "ae",
date = AESTDTM
)
ae_end <- date_source(
dataset_name = "ae",
date = AEENDTM
)
ae_ext <- admiral_ae %>%
derive_vars_dtm(
dtc = AESTDTC,
new_vars_prefix = "AEST",
highest_imputation = "M"
) %>%
derive_vars_dtm(
dtc = AEENDTC,
new_vars_prefix = "AEEN",
highest_imputation = "M"
)
lb_date <- date_source(
dataset_name = "lb",
date = LBDTM,
filter = !is.na(LBDTM)
)
lb_ext <- derive_vars_dtm(
admiral_lb,
dtc = LBDTC,
new_vars_prefix = "LB"
)
adsl_date <- date_source(dataset_name = "adsl", date = TRTEDTM)
admiral_dm %>%
derive_var_extreme_dtm(
new_var = LSTALVDTM,
ae_start, ae_end, lb_date, adsl_date,
source_datasets = list(
adsl = admiral_adsl,
ae = ae_ext, lb = lb_ext
),
mode = "last"
) %>%
select(USUBJID, LSTALVDTM)
#> # A tibble: 306 x 2
#> USUBJID LSTALVDTM
#> <chr> <dttm>
#> 1 01-701-1015 2014-07-02 23:59:59
#> 2 01-701-1023 2012-09-02 10:15:00
#> 3 01-701-1028 2014-01-14 23:59:59
#> 4 01-701-1033 2014-04-14 10:38:00
#> 5 01-701-1034 2014-12-30 23:59:59
#> 6 01-701-1047 2013-04-07 11:20:00
#> 7 01-701-1057 NA
#> 8 01-701-1097 2014-07-09 23:59:59
#> 9 01-701-1111 2012-09-17 11:48:00
#> 10 01-701-1115 2013-01-23 23:59:59
#> # … with 296 more rows
# derive last alive datetime and traceability variables
ae_start <- date_source(
dataset_name = "ae",
date = AESTDTM,
traceability_vars = exprs(
LALVDOM = "AE",
LALVSEQ = AESEQ,
LALVVAR = "AESTDTC"
)
)
ae_end <- date_source(
dataset_name = "ae",
date = AEENDTM,
traceability_vars = exprs(
LALVDOM = "AE",
LALVSEQ = AESEQ,
LALVVAR = "AEENDTC"
)
)
lb_date <- date_source(
dataset_name = "lb",
date = LBDTM,
filter = !is.na(LBDTM),
traceability_vars = exprs(
LALVDOM = "LB",
LALVSEQ = LBSEQ,
LALVVAR = "LBDTC"
)
)
adsl_date <- date_source(
dataset_name = "adsl",
date = TRTEDTM,
traceability_vars = exprs(
LALVDOM = "ADSL",
LALVSEQ = NA_integer_,
LALVVAR = "TRTEDTM"
)
)
admiral_dm %>%
derive_var_extreme_dtm(
new_var = LSTALVDTM,
ae_start, ae_end, lb_date, adsl_date,
source_datasets = list(
adsl = admiral_adsl,
ae = ae_ext,
lb = lb_ext
),
mode = "last"
) %>%
select(USUBJID, LSTALVDTM, LALVDOM, LALVSEQ, LALVVAR)
#> # A tibble: 306 x 5
#> USUBJID LSTALVDTM LALVDOM LALVSEQ LALVVAR
#> <chr> <dttm> <chr> <dbl> <chr>
#> 1 01-701-1015 2014-07-02 23:59:59 ADSL NA TRTEDTM
#> 2 01-701-1023 2012-09-02 10:15:00 LB 107 LBDTC
#> 3 01-701-1028 2014-01-14 23:59:59 ADSL NA TRTEDTM
#> 4 01-701-1033 2014-04-14 10:38:00 LB 107 LBDTC
#> 5 01-701-1034 2014-12-30 23:59:59 ADSL NA TRTEDTM
#> 6 01-701-1047 2013-04-07 11:20:00 LB 134 LBDTC
#> 7 01-701-1057 NA NA NA NA
#> 8 01-701-1097 2014-07-09 23:59:59 ADSL NA TRTEDTM
#> 9 01-701-1111 2012-09-17 11:48:00 LB 73 LBDTC
#> 10 01-701-1115 2013-01-23 23:59:59 ADSL NA TRTEDTM
#> # … with 296 more rows