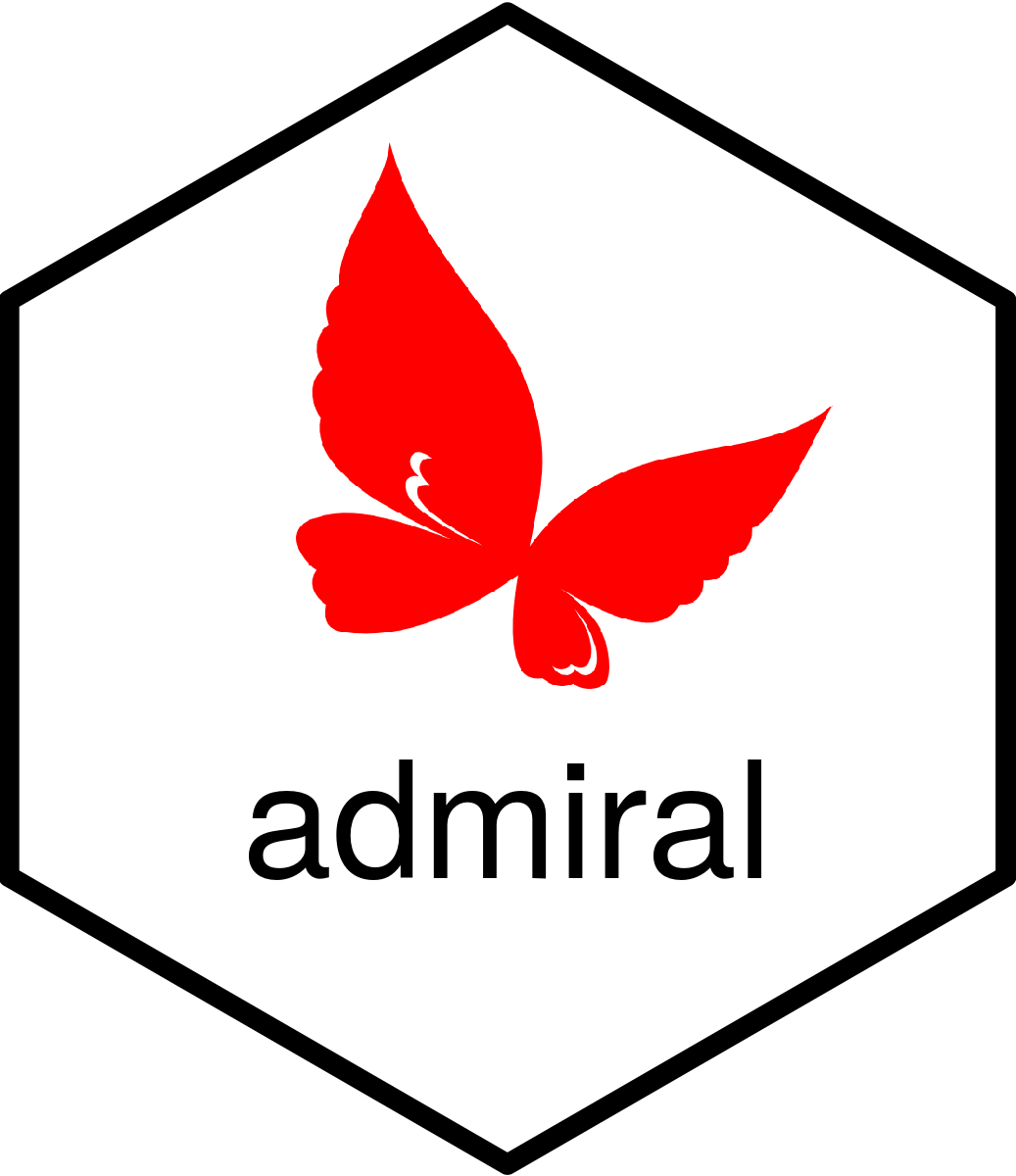
Add the Worst or Best Observation for Each By Group as New Records
Source:R/derive_extreme_event.R
derive_extreme_event.Rd
Add the first available record from events
for each by group as new
records, all variables of the selected observation are kept. It can be used
for selecting the extreme observation from a series of user-defined events.
This distinguish derive_extreme_event()
from derive_extreme_records()
,
where extreme records are derived based on certain order of existing
variables.
Usage
derive_extreme_event(
dataset,
by_vars = NULL,
events,
order,
mode,
check_type = "warning",
set_values_to
)
Arguments
- dataset
Input dataset
The variables specified by the
order
and theby_vars
parameter are expected.- by_vars
Grouping variables
Default:
NULL
Permitted Values: list of variables created by
exprs()
- events
Conditions and new values defining events
A list of
event()
objects is expected. Only observations listed in theevents
are considered for deriving extreme event. If multiple records meet the filtercondition
, take the first record sorted byorder
.- order
Sort order
If a particular event from
events
has more than one observation, within the event and by group, the records are ordered by the specified order.Permitted Values: list of variables or
desc(<variable>)
function calls created byexprs()
, e.g.,exprs(ADT, desc(AVAL))
- mode
Selection mode (first or last)
If a particular event from
events
has more than one observation, "first"/"last" is to select the first/last record of this type of events sorting byorder
.Permitted Values:
"first"
,"last"
- check_type
Check uniqueness?
If
"warning"
or"error"
is specified, the specified message is issued if the observations of the input dataset are not unique with respect to the by variables and the order.Default:
"warning"
Permitted Values:
"none"
,"warning"
,"error"
- set_values_to
Variables to be set
The specified variables are set to the specified values for the new observations.
A list of variable name-value pairs is expected.
LHS refers to a variable.
RHS refers to the values to set to the variable. This can be a string, a symbol, a numeric value or
NA
, e.g.,exprs(PARAMCD = "TDOSE", PARCAT1 = "OVERALL")
. More general expression are not allowed.
Value
The input dataset with the best or worst observation of each by group added as new observations.
Details
Construct a dataset based on
events
: apply the filtercondition
andset_values_to
to the input dataset.For each group (with respect to the variables specified for the
by_vars
parameter) the first or last observation (with respect to the order specified for theorder
parameter and the mode specified for themode
parameter) is selected.The variables specified by the
set_values_to
parameter are added to the selected observations.The observations are added to input dataset.
See also
BDS-Findings Functions for adding Parameters/Records:
default_qtc_paramcd()
,
derive_expected_records()
,
derive_extreme_records()
,
derive_locf_records()
,
derive_param_bmi()
,
derive_param_bsa()
,
derive_param_computed()
,
derive_param_doseint()
,
derive_param_exist_flag()
,
derive_param_exposure()
,
derive_param_extreme_event()
,
derive_param_framingham()
,
derive_param_map()
,
derive_param_qtc()
,
derive_param_rr()
,
derive_param_wbc_abs()
,
derive_summary_records()
Examples
library(tibble)
adqs <- tribble(
~USUBJID, ~PARAMCD, ~AVALC, ~ADY,
"1", "NO SLEEP", "N", 1,
"1", "WAKE UP", "N", 2,
"1", "FALL ASLEEP", "N", 3,
"2", "NO SLEEP", "N", 1,
"2", "WAKE UP", "Y", 2,
"2", "WAKE UP", "Y", 3,
"2", "FALL ASLEEP", "N", 4,
"3", "NO SLEEP", NA_character_, 1
)
# Add a new record for each USUBJID storing the the worst sleeping problem.
derive_extreme_event(
adqs,
by_vars = exprs(USUBJID),
events = list(
event(
condition = PARAMCD == "NO SLEEP" & AVALC == "Y",
set_values_to = exprs(AVALC = "No sleep", AVAL = 1)
),
event(
condition = PARAMCD == "WAKE UP" & AVALC == "Y",
set_values_to = exprs(AVALC = "Waking up more than three times", AVAL = 2)
),
event(
condition = PARAMCD == "FALL ASLEEP" & AVALC == "Y",
set_values_to = exprs(AVALC = "More than 30 mins to fall asleep", AVAL = 3)
),
event(
condition = all(AVALC == "N"),
set_values_to = exprs(
AVALC = "No sleeping problems", AVAL = 4
)
),
event(
condition = TRUE,
set_values_to = exprs(AVALC = "Missing", AVAL = 99)
)
),
order = exprs(ADY),
mode = "last",
set_values_to = exprs(
PARAMCD = "WSP",
PARAM = "Worst Sleeping Problems"
)
)
#> # A tibble: 11 x 6
#> USUBJID PARAMCD AVALC ADY AVAL PARAM
#> <chr> <chr> <chr> <dbl> <dbl> <chr>
#> 1 1 NO SLEEP N 1 NA NA
#> 2 1 WAKE UP N 2 NA NA
#> 3 1 FALL ASLEEP N 3 NA NA
#> 4 2 NO SLEEP N 1 NA NA
#> 5 2 WAKE UP Y 2 NA NA
#> 6 2 WAKE UP Y 3 NA NA
#> 7 2 FALL ASLEEP N 4 NA NA
#> 8 3 NO SLEEP NA 1 NA NA
#> 9 1 WSP No sleeping problems 3 4 Worst Sleeping Pro…
#> 10 2 WSP Waking up more than thre… 3 2 Worst Sleeping Pro…
#> 11 3 WSP Missing 1 99 Worst Sleeping Pro…